This repository was archived by the owner on Apr 12, 2024. It is now read-only.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
I've been profiling a one page app using Angular.js and have had a tough time nailing down this memory leak. I'm using Angularjs for a custom template system that outputs data from a content DSL containing educational content. In this system I swap out a lot of templates full of other angular directives which in themselves sometimes compile/transclude content. After a few days of searching I've finally narrowed down one of the larger memory leaks. Using
transclude: true
in a directive is fine, no problems with leaks, but as soon asng-include
is used within the template I was getting a lot of detached DOM fragments in the Chrome memory profiler.Here is a sample app which replicates the problem:
So running this using
ng-transclude
in thesecondLevel
directive, you can click the buttons a few times to see the transclude working, but when profiled in dev tools we get:As you can see there are 4 detached Dom node Trees as a result, not a huge deal in this smaller app, but in my larger app it's causing 350+ detached nodes and about 5mb heap increase per template change. This is a fairly large problem for a one page app since we never reload the page.
Now if we swap out
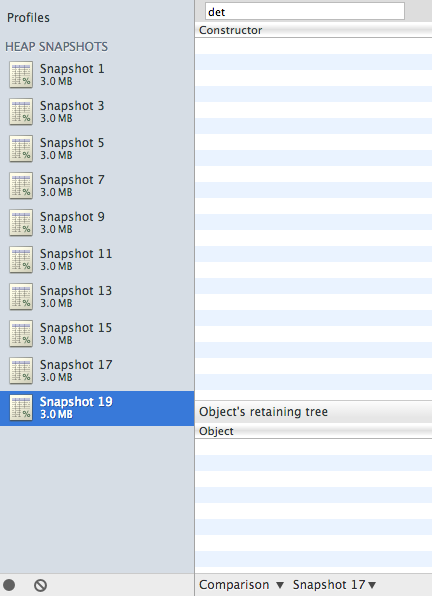
ng-transclude
with the proposed changes (ng-transclude-fix
) we get:No detached dom nodes!
The tests pass, I'm not entirely sure how you would even test for detached Dom nodes and memory heap size. From my testing, it seems like the act of assigning $transclude to the controller (or scope for that matter) causes the detached nodes. This fix uses the new transclude function included within the
link
function to handle the transclude, instead of passing the $transclude object from the controller to the linking function. This is functionally the same, and eliminates the leak.