|
| 1 | +# 1719. Number Of Ways To Reconstruct A Tree |
| 2 | + |
| 3 | +- Difficulty: Hard. |
| 4 | +- Related Topics: Tree, Graph. |
| 5 | +- Similar Questions: Create Binary Tree From Descriptions, Maximum Star Sum of a Graph. |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +You are given an array `pairs`, where `pairs[i] = [xi, yi]`, and: |
| 10 | + |
| 11 | + |
| 12 | + |
| 13 | +- There are no duplicates. |
| 14 | + |
| 15 | +- `xi < yi` |
| 16 | + |
| 17 | + |
| 18 | +Let `ways` be the number of rooted trees that satisfy the following conditions: |
| 19 | + |
| 20 | + |
| 21 | + |
| 22 | +- The tree consists of nodes whose values appeared in `pairs`. |
| 23 | + |
| 24 | +- A pair `[xi, yi]` exists in `pairs` **if and only if** `xi` is an ancestor of `yi` or `yi` is an ancestor of `xi`. |
| 25 | + |
| 26 | +- **Note:** the tree does not have to be a binary tree. |
| 27 | + |
| 28 | + |
| 29 | +Two ways are considered to be different if there is at least one node that has different parents in both ways. |
| 30 | + |
| 31 | +Return: |
| 32 | + |
| 33 | + |
| 34 | + |
| 35 | +- `0` if `ways == 0` |
| 36 | + |
| 37 | +- `1` if `ways == 1` |
| 38 | + |
| 39 | +- `2` if `ways > 1` |
| 40 | + |
| 41 | + |
| 42 | +A **rooted tree** is a tree that has a single root node, and all edges are oriented to be outgoing from the root. |
| 43 | + |
| 44 | +An **ancestor** of a node is any node on the path from the root to that node (excluding the node itself). The root has no ancestors. |
| 45 | + |
| 46 | + |
| 47 | +Example 1: |
| 48 | + |
| 49 | +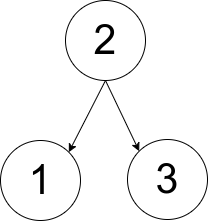 |
| 50 | + |
| 51 | +``` |
| 52 | +Input: pairs = [[1,2],[2,3]] |
| 53 | +Output: 1 |
| 54 | +Explanation: There is exactly one valid rooted tree, which is shown in the above figure. |
| 55 | +``` |
| 56 | + |
| 57 | +Example 2: |
| 58 | + |
| 59 | +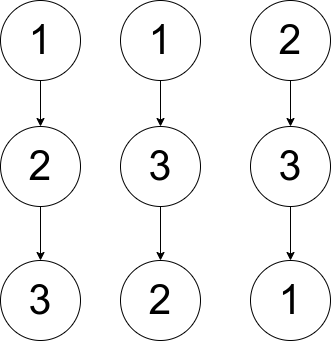 |
| 60 | + |
| 61 | +``` |
| 62 | +Input: pairs = [[1,2],[2,3],[1,3]] |
| 63 | +Output: 2 |
| 64 | +Explanation: There are multiple valid rooted trees. Three of them are shown in the above figures. |
| 65 | +``` |
| 66 | + |
| 67 | +Example 3: |
| 68 | + |
| 69 | +``` |
| 70 | +Input: pairs = [[1,2],[2,3],[2,4],[1,5]] |
| 71 | +Output: 0 |
| 72 | +Explanation: There are no valid rooted trees. |
| 73 | +``` |
| 74 | + |
| 75 | + |
| 76 | +**Constraints:** |
| 77 | + |
| 78 | + |
| 79 | + |
| 80 | +- `1 <= pairs.length <= 105` |
| 81 | + |
| 82 | +- `1 <= xi < yi <= 500` |
| 83 | + |
| 84 | +- The elements in `pairs` are unique. |
| 85 | + |
| 86 | + |
| 87 | + |
| 88 | +## Solution |
| 89 | + |
| 90 | +```javascript |
| 91 | +/** |
| 92 | + * @param {number[][]} pairs |
| 93 | + * @return {number} |
| 94 | + */ |
| 95 | +var checkWays = function(pairs) { |
| 96 | + var map = {}; |
| 97 | + for (var i = 0; i < pairs.length; i++) { |
| 98 | + var [x, y] = pairs[i]; |
| 99 | + if (!map[x]) map[x] = []; |
| 100 | + if (!map[y]) map[y] = []; |
| 101 | + map[x].push(y); |
| 102 | + map[y].push(x); |
| 103 | + } |
| 104 | + var nums = Object.keys(map).sort((a, b) => map[a].length - map[b].length); |
| 105 | + var visited = {}; |
| 106 | + var result = 1; |
| 107 | + for (var i = nums.length - 1; i >= 0; i--) { |
| 108 | + var num = nums[i]; |
| 109 | + var parentDegree = Number.MAX_SAFE_INTEGER; |
| 110 | + var parent = -1; |
| 111 | + for (var j = 0; j < map[num].length; j++) { |
| 112 | + var n = map[num][j]; |
| 113 | + if (visited[n] && map[n].length >= map[num].length && map[n].length < parentDegree) { |
| 114 | + parentDegree = map[n].length; |
| 115 | + parent = n; |
| 116 | + } |
| 117 | + } |
| 118 | + visited[num] = true; |
| 119 | + if (parent === -1) { |
| 120 | + if (map[num].length === nums.length - 1) continue; |
| 121 | + return 0; |
| 122 | + } |
| 123 | + for (var j = 0; j < map[num].length; j++) { |
| 124 | + if (map[num][j] !== parent && !map[parent].includes(map[num][j])) { |
| 125 | + return 0; |
| 126 | + } |
| 127 | + } |
| 128 | + if (map[parent].length === map[num].length) { |
| 129 | + result = 2; |
| 130 | + } |
| 131 | + } |
| 132 | + return result; |
| 133 | +}; |
| 134 | +``` |
| 135 | + |
| 136 | +**Explain:** |
| 137 | + |
| 138 | +see https://leetcode.com/problems/number-of-ways-to-reconstruct-a-tree/solutions/1009393/c-o-nlogn-soln-with-comments-descriptive-variable-naming-time-space-complexity-analysis/ |
| 139 | + |
| 140 | +**Complexity:** |
| 141 | + |
| 142 | +* Time complexity : O(n ^ 2). |
| 143 | +* Space complexity : O(n ^ 2). |
0 commit comments