{{ post.data.title }}
@@ -189,22 +190,21 @@ Display the results ``` -Here's a preview: +预览效果如下: 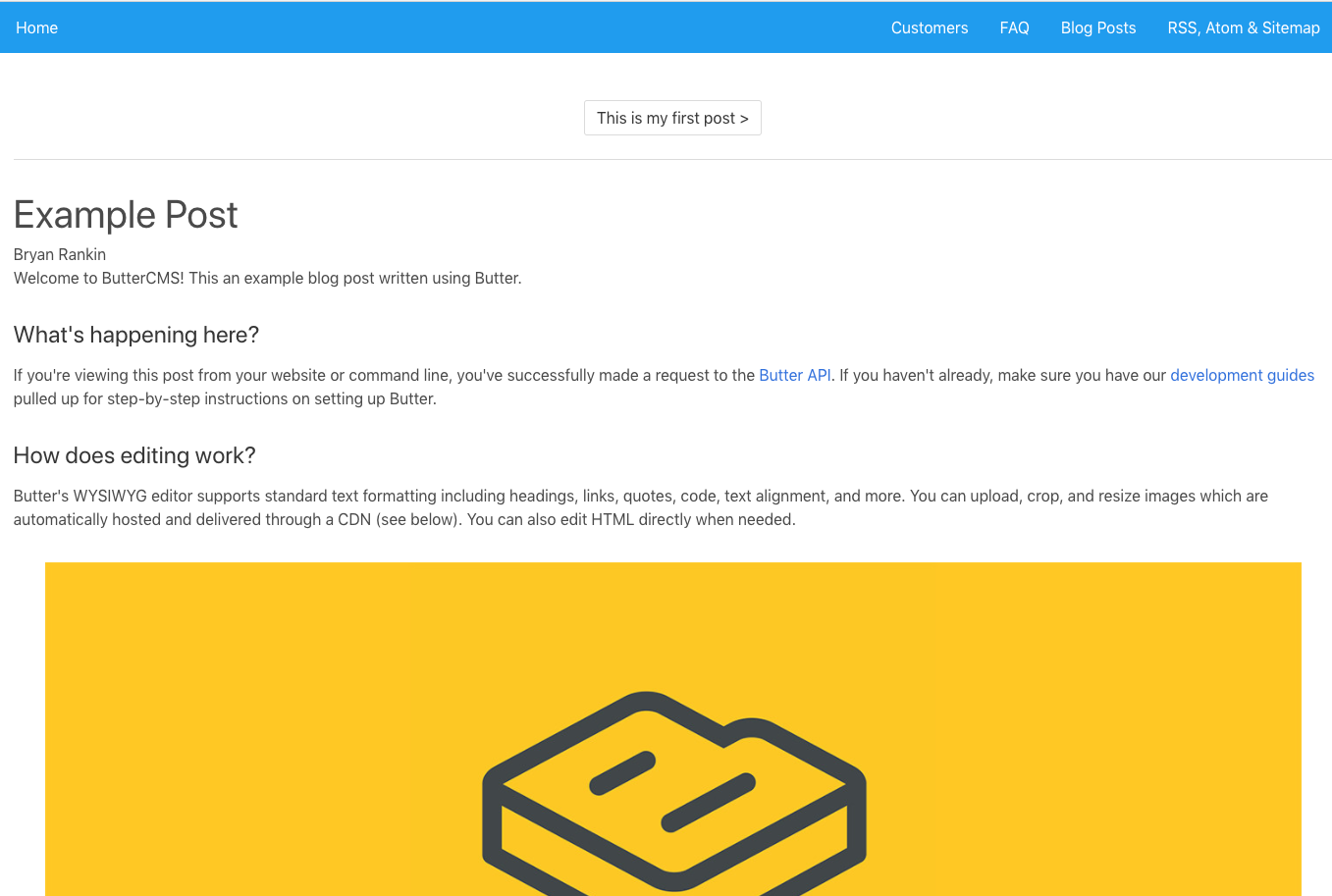 +现在我们的应用已经拉取了所有博文并且可以导航到每个独立的博文。但上一篇博文/下一篇博文的链接还不工作。 -Now our app is pulling all blog posts and we can navigate to individual posts. However, our next/previous post buttons are not working. +需要注意的一点是在通过参数控制路由时,比如当用户从 `/blog/foo` 导航至 `/blog/bar` 时,我们复用了相同的组件实例。因为这两个路由渲染了相同的组件,所以比销毁老实例再创建新实例的效率更高。 -One thing to note when using routes with params is that when the user navigates from /blog/foo to /blog/bar, the same component instance will be reused. Since both routes render the same component, this is more efficient than destroying the old instance and then creating a new one. +注意,用这种方式使用组件意味着这个组件的生命周期钩子将不会被调用。请移步 Vue Router 的文档了解[动态路由匹配](https://router.vuejs.org/en/essentials/dynamic-matching.html)。
-Be aware, that using the component this way will mean that the lifecycle hooks of the component will not be called. Visit the Vue.js docs to learn more about [Dynamic Route Matching](https://router.vuejs.org/en/essentials/dynamic-matching.html)
+为了解决这个问题,我们需要匹配 `$route` 对象并在路由发生变化的时候调用 `getPost()`。 -To fix this we need to watch the `$route` object and call `getPost()` when the route changes. +更新 `components/BlogPost.vue` 中的 ` ``` -Now your app has a working blog that can be updated easily in the ButterCMS dashboard. +现在你的应用就有了可工作的博客,你可以在 ButterCMS 仪表盘便捷地更新它。 -## Categories, Tags, and Authors +## 分类、标签和作者 -Use Butter's APIs for categories, tags, and authors to feature and filter content on your blog. +使用 Butter 关于分类、标签和作者的 API 来设置和过滤你的博客。 -See the ButterCMS API reference for more information about these objects: +你可以移步到 ButterCMS API 参考文档来进一步了解这些对象: * [Categories](https://buttercms.com/docs/api/?ruby#categories) * [Tags](https://buttercms.com/docs/api/?ruby#tags) * [Authors](https://buttercms.com/docs/api/?ruby#authors) -Here's an example of listing all categories and getting posts by category. Call these methods on the `created()` lifecycle hook: +这里有一个示例,列出所有分类并根据分类获取博文列表。在生命周期钩子 `created()` 中调用这些方法: -``` +```javascript methods: { ... getCategories() { @@ -278,12 +277,10 @@ created() { } ``` -## Alternative Patterns - -An alternative pattern to consider, especially if you prefer writing only in Markdown, is using something like [Nuxtent](https://nuxtent.now.sh/guide/writing#async-components). Nuxtent allows you to use `Vue Component` inside of Markdown files. This approach would be akin to a static site approach (i.e. Jekyll) where you compose your blog posts in Markdown files. Nuxtent adds a nice integration between Vue.js and Markdown allowing you to live in a 100% Vue.js world. - +## 替代方案 -## Wrap up +有一个替代方案,尤其在你只喜欢写 Markdown 时适用,就是使用诸如 [Nuxtent](https://nuxtent.now.sh/guide/writing#async-components) 的工具。Nuxtent 允许你在 Markdown 文件内部使用 `Vue Component`。它类似一个静态站点工具 (例如 Jekyll),让你在 Markdown 文件中撰写你的博文。Nuxtent 将 Vue.js 和 Markdown 很好地集成了起来,让你完全生活在 Vue.js 的世界里。 -That's it! You now have a fully functional CMS-powered blog running in your app. We hope this tutorial was helpful and made your development experience with Vue.js even more enjoyable :) +## 总结 +差不多就是这些了!现在你已经在自己的应用中拥有了一个可以正常工作的 CMS 博客。我们希望这份教程可以帮助你,使你的 Vue.js 开发体验更有乐趣 :)