diff --git a/README.md b/README.md
index e45df6e..28b64d2 100644
--- a/README.md
+++ b/README.md
@@ -3,10 +3,8 @@
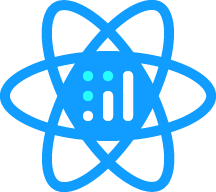
> A [plotly.js](https://github.com/plotly/plotly.js) React component from
-> [Plotly](https://plot.ly/). The basis of Plotly's
-> [React component suite](https://plot.ly/products/react/).
-
-👉 [DEMO](http://react-plotly.js-demo.getforge.io/)
+> [Plotly](https://plotly.com/). The basis of Plotly's
+> [React component suite](https://plotly.com/products/react/).
👉 [Demo source code](https://github.com/plotly/react-plotly.js-demo-app)
@@ -28,7 +26,7 @@
## Installation
```bash
-$ npm install react-plotly.js plotly.js
+$ npm install react-plotly.js plotly.js-dist
```
## Quick start
@@ -68,8 +66,8 @@ You should see a plot like this:
For a full description of Plotly chart types and attributes see the following resources:
-- [Plotly JavaScript API documentation](https://plot.ly/javascript/)
-- [Full plotly.js attribute listing](https://plot.ly/javascript/reference/)
+- [Plotly JavaScript API documentation](https://plotly.com/javascript/)
+- [Full plotly.js attribute listing](https://plotly.com/javascript/reference/)
## State management
@@ -101,15 +99,15 @@ class App extends React.Component {
## Refreshing the Plot
-This component will refresh the plot via [`Plotly.react`](https://plot.ly/javascript/plotlyjs-function-reference/#plotlyreact) if any of the following are true:
+This component will refresh the plot via [`Plotly.react`](https://plotly.com/javascript/plotlyjs-function-reference/#plotlyreact) if any of the following are true:
- The `revision` prop is defined and has changed, OR;
- One of `data`, `layout` or `config` has changed identity as checked via a shallow `===`, OR;
- The number of elements in `frames` has changed
-Furthermore, when called, [`Plotly.react`](https://plot.ly/javascript/plotlyjs-function-reference/#plotlyreact) will only refresh the data being plotted if the _identity_ of the data arrays (e.g. `x`, `y`, `marker.color` etc) has changed, or if `layout.datarevision` has changed.
+Furthermore, when called, [`Plotly.react`](https://plotly.com/javascript/plotlyjs-function-reference/#plotlyreact) will only refresh the data being plotted if the _identity_ of the data arrays (e.g. `x`, `y`, `marker.color` etc) has changed, or if `layout.datarevision` has changed.
-In short, this means that simply adding data points to a trace in `data` or changing a value in `layout` will not cause a plot to update unless this is done immutably via something like [immutability-helper](https://github.com/kolodny/immutability-helper) if performance considerations permit it, or unless `revision` and/or [`layout.datarevision`](https://plot.ly/javascript/reference/#layout-datarevision) are used to force a rerender.
+In short, this means that simply adding data points to a trace in `data` or changing a value in `layout` will not cause a plot to update unless this is done immutably via something like [immutability-helper](https://github.com/kolodny/immutability-helper) if performance considerations permit it, or unless `revision` and/or [`layout.datarevision`](https://plotly.com/javascript/reference/#layout-datarevision) are used to force a rerender.
## API Reference
@@ -119,10 +117,10 @@ In short, this means that simply adding data points to a trace in `data` or chan
| Prop | Type | Default | Description |
| ------------------ | ---------------------------- | ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `data` | `Array` | `[]` | list of trace objects (see https://plot.ly/javascript/reference/) |
-| `layout` | `Object` | `undefined` | layout object (see https://plot.ly/javascript/reference/#layout) |
-| `frames` | `Array` | `undefined` | list of frame objects (see https://plot.ly/javascript/reference/) |
-| `config` | `Object` | `undefined` | config object (see https://plot.ly/javascript/configuration-options/) |
+| `data` | `Array` | `[]` | list of trace objects (see https://plotly.com/javascript/reference/) |
+| `layout` | `Object` | `undefined` | layout object (see https://plotly.com/javascript/reference/#layout) |
+| `frames` | `Array` | `undefined` | list of frame objects (see https://plotly.com/javascript/reference/) |
+| `config` | `Object` | `undefined` | config object (see https://plotly.com/javascript/configuration-options/) |
| `revision` | `Number` | `undefined` | When provided, causes the plot to update when the revision is incremented. |
| `onInitialized` | `Function(figure, graphDiv)` | `undefined` | Callback executed after plot is initialized. See below for parameter information. |
| `onUpdate` | `Function(figure, graphDiv)` | `undefined` | Callback executed when a plot is updated due to new data or layout, or when user interacts with a plot. See below for parameter information. |
@@ -134,7 +132,7 @@ In short, this means that simply adding data points to a trace in `data` or chan
| `debug` | `Boolean` | `false` | Assign the graph div to `window.gd` for debugging |
| `useResizeHandler` | `Boolean` | `false` | When true, adds a call to `Plotly.Plot.resize()` as a `window.resize` event handler |
-**Note**: To make a plot responsive, i.e. to fill its containing element and resize when the window is resized, use `style` or `className` to set the dimensions of the element (i.e. using `width: 100%; height: 100%` or some similar values) and set `useResizeHandler` to `true` while setting `layout.autosize` to `true` and leaving `layout.height` and `layout.width` undefined. This can be seen in action in [this CodePen](https://codepen.io/nicolaskruchten/pen/ERgBZX) and will implement the behaviour documented here: https://plot.ly/javascript/responsive-fluid-layout/
+**Note**: To make a plot responsive, i.e. to fill its containing element and resize when the window is resized, use `style` or `className` to set the dimensions of the element (i.e. using `width: 100%; height: 100%` or some similar values) and set `useResizeHandler` to `true` while setting `layout.autosize` to `true` and leaving `layout.height` and `layout.width` undefined. This can be seen in action in [this CodePen](https://codepen.io/nicolaskruchten/pen/ERgBZX) and will implement the behaviour documented here: https://plotly.com/javascript/responsive-fluid-layout/
#### Callback signature: `Function(figure, graphDiv)`
@@ -146,7 +144,7 @@ The `onInitialized`, `onUpdate` and `onPurge` props are all functions which will
### Event handler props
-Event handlers for specific [`plotly.js` events](https://plot.ly/javascript/plotlyjs-events/) may be attached through the following props:
+Event handlers for specific [`plotly.js` events](https://plotly.com/javascript/plotlyjs-events/) may be attached through the following props:
| Prop | Type | Plotly Event |
| ------------------------- | ---------- | ------------------------------ |
@@ -186,7 +184,7 @@ Event handlers for specific [`plotly.js` events](https://plot.ly/javascript/plot
By default, the `Plot` component exported by this library loads a precompiled version of all of `plotly.js`, so `plotly.js` must be installed as a peer dependency. This bundle is around 6Mb unminified, and minifies to just over 2Mb.
-If you do not wish to use this version of `plotly.js`, e.g. if you want to use a [different precompiled bundle](https://github.com/plotly/plotly.js/blob/master/dist/README.md#partial-bundles) or if your wish to [assemble you own customized bundle](https://github.com/plotly/plotly.js/blob/master/CUSTOM_BUNDLE.md), or if you wish to load `plotly.js` [from a CDN](https://github.com/plotly/plotly.js#use-the-plotlyjs-cdn-hosted-by-fastly), you can skip the installation of as a peer dependency (and ignore the resulting warning) and use the `createPlotComponent` method to get a `Plot` component, instead of importing it:
+If you do not wish to use this version of `plotly.js`, e.g. if you want to use a [different precompiled bundle](https://github.com/plotly/plotly.js/blob/master/dist/README.md#partial-bundles) or if your wish to [assemble you own customized bundle](https://github.com/plotly/plotly.js/blob/master/CUSTOM_BUNDLE.md), or if you wish to load `plotly.js` [from a CDN](https://github.com/plotly/plotly.js#load-via-script-tag), you can skip the installation of as a peer dependency (and ignore the resulting warning) and use the `createPlotComponent` method to get a `Plot` component, instead of importing it:
```javascript
// simplest method: uses precompiled complete bundle from `plotly.js`
@@ -204,7 +202,7 @@ For quick one-off demos on [CodePen](https://codepen.io/) or [JSFiddle](https://
You can load plotly.js and the component factory with:
```html
-
+
```
diff --git a/package.json b/package.json
index 945785a..2f1835b 100644
--- a/package.json
+++ b/package.json
@@ -58,7 +58,7 @@
"mkdirp": "^1.0.4",
"nodemon": "^2.0.6",
"onetime": "^5.1.2",
- "plotly.js": "^1.35.0",
+ "plotly.js-dist": "^2.0.0",
"prettier": "^2.2.1",
"react": "^16.13.1",
"react-addons-test-utils": "^15.6.0",
@@ -69,7 +69,7 @@
"uglify-js": "^3.12.1"
},
"peerDependencies": {
- "plotly.js": ">1.34.0",
+ "plotly.js-dist": ">=2.0.0",
"react": ">0.13.0"
},
"browserify-global-shim": {
diff --git a/src/__mocks__/plotly.js b/src/__mocks__/plotly.js
index fe1ad31..0cecc1e 100644
--- a/src/__mocks__/plotly.js
+++ b/src/__mocks__/plotly.js
@@ -4,12 +4,6 @@ const state = {};
const ASYNC_DELAY = 1;
export default {
- plot: jest.fn((gd) => {
- state.gd = gd;
- setTimeout(() => {
- state.gd.emit('plotly_afterplot');
- }, ASYNC_DELAY);
- }),
newPlot: jest.fn((gd) => {
state.gd = gd;
EventEmitter(state.gd); // eslint-disable-line new-cap
diff --git a/src/react-plotly.js b/src/react-plotly.js
index ac2794a..60ee933 100644
--- a/src/react-plotly.js
+++ b/src/react-plotly.js
@@ -1,5 +1,5 @@
import plotComponentFactory from './factory';
-import Plotly from 'plotly.js/dist/plotly';
+import Plotly from 'plotly.js-dist';
const PlotComponent = plotComponentFactory(Plotly);