Commit 5ff5584
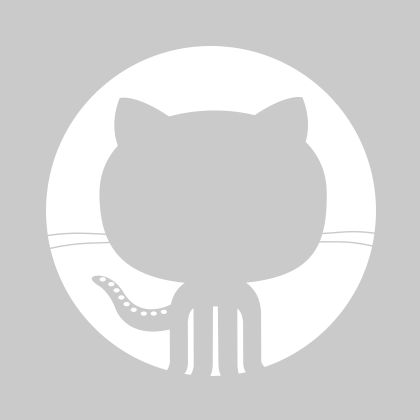
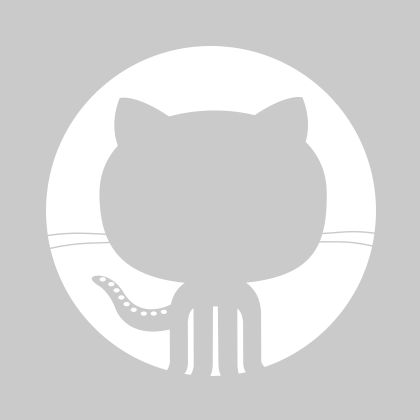
Terji Petersen
Terji Petersen
1 parent 0b93117 commit 5ff5584
File tree
33 files changed
+255
-289
lines changed- pandas
- core
- indexes
- internals
- tests
- apply
- arrays
- interval
- sparse
- frame
- indexing
- methods
- indexes
- datetimes
- multi
- numeric
- ranges
- timedeltas
- io
- pytables
- reshape
- merge
- series
- accessors
- indexing
- util
33 files changed
+255
-289
lines changedLines changed: 0 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
593 | 593 |
| |
594 | 594 |
| |
595 | 595 |
| |
596 |
| - | |
597 |
| - | |
598 | 596 |
| |
599 |
| - | |
600 | 597 |
| |
601 | 598 |
| |
602 | 599 |
| |
|
Lines changed: 41 additions & 27 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
89 | 89 |
| |
90 | 90 |
| |
91 | 91 |
| |
| 92 | + | |
92 | 93 |
| |
93 | 94 |
| |
94 | 95 |
| |
| |||
104 | 105 |
| |
105 | 106 |
| |
106 | 107 |
| |
| 108 | + | |
107 | 109 |
| |
108 | 110 |
| |
109 | 111 |
| |
| |||
588 | 590 |
| |
589 | 591 |
| |
590 | 592 |
| |
591 |
| - | |
592 |
| - | |
593 |
| - | |
594 |
| - | |
595 |
| - | |
596 |
| - | |
597 |
| - | |
598 |
| - | |
599 |
| - | |
600 |
| - | |
| 593 | + | |
| 594 | + | |
| 595 | + | |
| 596 | + | |
| 597 | + | |
| 598 | + | |
601 | 599 |
| |
602 |
| - | |
| 600 | + | |
603 | 601 |
| |
604 | 602 |
| |
605 | 603 |
| |
| |||
1040 | 1038 |
| |
1041 | 1039 |
| |
1042 | 1040 |
| |
1043 |
| - | |
1044 |
| - | |
1045 |
| - | |
| 1041 | + | |
| 1042 | + | |
| 1043 | + | |
| 1044 | + | |
| 1045 | + | |
1046 | 1046 |
| |
1047 | 1047 |
| |
1048 |
| - | |
1049 |
| - | |
| 1048 | + | |
| 1049 | + | |
| 1050 | + | |
| 1051 | + | |
1050 | 1052 |
| |
| 1053 | + | |
| 1054 | + | |
| 1055 | + | |
| 1056 | + | |
| 1057 | + | |
| 1058 | + | |
| 1059 | + | |
| 1060 | + | |
| 1061 | + | |
| 1062 | + | |
| 1063 | + | |
1051 | 1064 |
| |
1052 | 1065 |
| |
1053 | 1066 |
| |
| |||
5247 | 5260 |
| |
5248 | 5261 |
| |
5249 | 5262 |
| |
| 5263 | + | |
5250 | 5264 |
| |
5251 | 5265 |
| |
5252 | 5266 |
| |
| |||
6115 | 6129 |
| |
6116 | 6130 |
| |
6117 | 6131 |
| |
6118 |
| - | |
6119 |
| - | |
6120 |
| - | |
6121 |
| - | |
6122 |
| - | |
6123 |
| - | |
6124 |
| - | |
6125 | 6132 |
| |
6126 | 6133 |
| |
6127 | 6134 |
| |
| |||
6598 | 6605 |
| |
6599 | 6606 |
| |
6600 | 6607 |
| |
6601 |
| - | |
6602 |
| - | |
6603 |
| - | |
6604 |
| - | |
| 6608 | + | |
| 6609 | + | |
| 6610 | + | |
| 6611 | + | |
| 6612 | + | |
| 6613 | + | |
| 6614 | + | |
| 6615 | + | |
| 6616 | + | |
| 6617 | + | |
| 6618 | + | |
6605 | 6619 |
| |
6606 | 6620 |
| |
6607 | 6621 |
| |
|
Lines changed: 29 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
| 16 | + | |
16 | 17 |
| |
17 | 18 |
| |
18 | 19 |
| |
| |||
174 | 175 |
| |
175 | 176 |
| |
176 | 177 |
| |
| 178 | + | |
| 179 | + | |
| 180 | + | |
| 181 | + | |
177 | 182 |
| |
178 | 183 |
| |
179 | 184 |
| |
| |||
198 | 203 |
| |
199 | 204 |
| |
200 | 205 |
| |
| 206 | + | |
| 207 | + | |
| 208 | + | |
201 | 209 |
| |
202 | 210 |
| |
203 | 211 |
| |
| |||
347 | 355 |
| |
348 | 356 |
| |
349 | 357 |
| |
350 |
| - | |
| 358 | + | |
| 359 | + | |
| 360 | + | |
| 361 | + | |
| 362 | + | |
| 363 | + | |
| 364 | + | |
| 365 | + | |
| 366 | + | |
| 367 | + | |
| 368 | + | |
| 369 | + | |
| 370 | + | |
| 371 | + | |
| 372 | + | |
| 373 | + | |
| 374 | + | |
| 375 | + | |
| 376 | + | |
| 377 | + | |
351 | 378 |
| |
352 | 379 |
| |
353 | 380 |
| |
| |||
391 | 418 |
| |
392 | 419 |
| |
393 | 420 |
| |
394 |
| - | |
| 421 | + | |
395 | 422 |
| |
396 | 423 |
| |
397 | 424 |
| |
|
Lines changed: 2 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
185 | 185 |
| |
186 | 186 |
| |
187 | 187 |
| |
188 |
| - | |
| 188 | + | |
189 | 189 |
| |
190 |
| - | |
| 190 | + | |
191 | 191 |
| |
192 | 192 |
| |
193 | 193 |
| |
|
Lines changed: 2 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
64 | 64 |
| |
65 | 65 |
| |
66 | 66 |
| |
67 |
| - | |
68 | 67 |
| |
69 | 68 |
| |
70 | 69 |
| |
| |||
1582 | 1581 |
| |
1583 | 1582 |
| |
1584 | 1583 |
| |
1585 |
| - | |
| 1584 | + | |
1586 | 1585 |
| |
1587 | 1586 |
| |
1588 | 1587 |
| |
| |||
1610 | 1609 |
| |
1611 | 1610 |
| |
1612 | 1611 |
| |
1613 |
| - | |
| 1612 | + | |
1614 | 1613 |
| |
1615 | 1614 |
| |
1616 | 1615 |
| |
|
Lines changed: 2 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
186 | 186 |
| |
187 | 187 |
| |
188 | 188 |
| |
189 |
| - | |
| 189 | + | |
190 | 190 |
| |
191 | 191 |
| |
192 | 192 |
| |
| |||
766 | 766 |
| |
767 | 767 |
| |
768 | 768 |
| |
769 |
| - | |
| 769 | + | |
770 | 770 |
| |
771 | 771 |
| |
772 | 772 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
287 | 287 |
| |
288 | 288 |
| |
289 | 289 |
| |
290 |
| - | |
| 290 | + | |
291 | 291 |
| |
292 | 292 |
| |
293 | 293 |
| |
|
Lines changed: 15 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
44 |
| - | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
45 | 50 |
| |
46 | 51 |
| |
47 | 52 |
| |
| |||
212 | 217 |
| |
213 | 218 |
| |
214 | 219 |
| |
215 |
| - | |
| 220 | + | |
| 221 | + | |
| 222 | + | |
| 223 | + | |
| 224 | + | |
| 225 | + | |
| 226 | + | |
| 227 | + | |
| 228 | + | |
216 | 229 |
| |
217 | 230 |
| |
218 | 231 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
736 | 736 |
| |
737 | 737 |
| |
738 | 738 |
| |
739 |
| - | |
| 739 | + | |
740 | 740 |
| |
741 | 741 |
| |
742 | 742 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
159 | 159 |
| |
160 | 160 |
| |
161 | 161 |
| |
162 |
| - | |
| 162 | + | |
163 | 163 |
| |
164 | 164 |
| |
165 | 165 |
| |
|
Lines changed: 2 additions & 6 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
835 | 835 |
| |
836 | 836 |
| |
837 | 837 |
| |
838 |
| - | |
839 |
| - | |
840 |
| - | |
841 |
| - | |
842 |
| - | |
| 838 | + | |
843 | 839 |
| |
844 | 840 |
| |
845 | 841 |
| |
| |||
850 | 846 |
| |
851 | 847 |
| |
852 | 848 |
| |
853 |
| - | |
| 849 | + | |
854 | 850 |
| |
855 | 851 |
| |
856 | 852 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
812 | 812 |
| |
813 | 813 |
| |
814 | 814 |
| |
815 |
| - | |
| 815 | + | |
816 | 816 |
| |
817 | 817 |
| |
818 | 818 |
| |
|
Lines changed: 2 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
281 | 281 |
| |
282 | 282 |
| |
283 | 283 |
| |
| 284 | + | |
284 | 285 |
| |
285 |
| - | |
| 286 | + | |
286 | 287 |
| |
287 | 288 |
| |
288 | 289 |
| |
|
0 commit comments