|
| 1 | +# 79. Word Search |
| 2 | +Given an `m x n` grid of characters `board` and a string `word`, return `true` *if* `word` *exists in the grid*. |
| 3 | + |
| 4 | +The word can be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once. |
| 5 | + |
| 6 | +#### Example 1: |
| 7 | +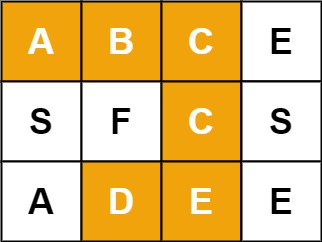 |
| 8 | +<pre> |
| 9 | +<strong>Input:</strong> board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED" |
| 10 | +<strong>Output:</strong> true |
| 11 | +</pre> |
| 12 | + |
| 13 | +#### Example 2: |
| 14 | +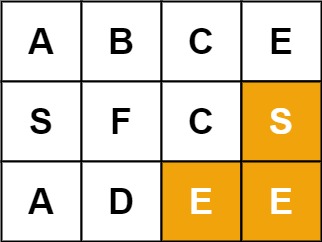 |
| 15 | +<pre> |
| 16 | +<strong>Input:</strong> board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "SEE" |
| 17 | +<strong>Output:</strong> true |
| 18 | +</pre> |
| 19 | + |
| 20 | +#### Example 3: |
| 21 | +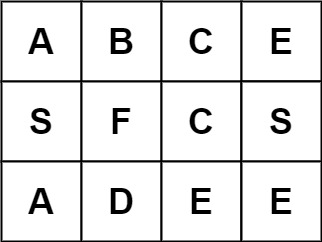 |
| 22 | +<pre> |
| 23 | +<strong>Input:</strong> board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCB" |
| 24 | +<strong>Output:</strong> false |
| 25 | +</pre> |
| 26 | + |
| 27 | +#### Constraints: |
| 28 | +* `m == board.length` |
| 29 | +* `n = board[i].length` |
| 30 | +* `1 <= m, n <= 6` |
| 31 | +* `1 <= word.length <= 15` |
| 32 | +* `board` and `word` consists of only lowercase and uppercase English letters. |
| 33 | + |
| 34 | +**Follow up:** Could you use search pruning to make your solution faster with a larger `board`? |
| 35 | + |
| 36 | +## Solutions (Python) |
| 37 | + |
| 38 | +### 1. Solution |
| 39 | +```Python |
| 40 | +class Solution: |
| 41 | + def exist(self, board: List[List[str]], word: str) -> bool: |
| 42 | + m, n = len(board), len(board[0]) |
| 43 | + |
| 44 | + for r in range(m): |
| 45 | + for c in range(n): |
| 46 | + if board[r][c] != word[0]: |
| 47 | + continue |
| 48 | + |
| 49 | + visited = {(r, c)} |
| 50 | + stack = [[r, c, 0, 0]] |
| 51 | + |
| 52 | + while stack != []: |
| 53 | + i, j, k, l = stack[-1] |
| 54 | + |
| 55 | + if k == len(word) - 1: |
| 56 | + return True |
| 57 | + |
| 58 | + if l == 0: |
| 59 | + stack[-1][3] += 1 |
| 60 | + if i > 0 and (i - 1, j) not in visited and board[i - 1][j] == word[k + 1]: |
| 61 | + visited.add((i - 1, j)) |
| 62 | + stack.append([i - 1, j, k + 1, 0]) |
| 63 | + elif l == 1: |
| 64 | + stack[-1][3] += 1 |
| 65 | + if i < m - 1 and (i + 1, j) not in visited and board[i + 1][j] == word[k + 1]: |
| 66 | + visited.add((i + 1, j)) |
| 67 | + stack.append([i + 1, j, k + 1, 0]) |
| 68 | + elif l == 2: |
| 69 | + stack[-1][3] += 1 |
| 70 | + if j > 0 and (i, j - 1) not in visited and board[i][j - 1] == word[k + 1]: |
| 71 | + visited.add((i, j - 1)) |
| 72 | + stack.append([i, j - 1, k + 1, 0]) |
| 73 | + elif l == 3: |
| 74 | + stack[-1][3] += 1 |
| 75 | + if j < n - 1 and (i, j + 1) not in visited and board[i][j + 1] == word[k + 1]: |
| 76 | + visited.add((i, j + 1)) |
| 77 | + stack.append([i, j + 1, k + 1, 0]) |
| 78 | + else: |
| 79 | + stack.pop() |
| 80 | + visited.remove((i, j)) |
| 81 | + |
| 82 | + return False |
| 83 | +``` |
0 commit comments