You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
The HTTP server always closes connections to it after 2s (HTTP_MAX_CLOSE_WAIT) and never accepts many requests from the same socket. It always returns the header Connection: close to tell the client to close the connection.
This is extremely inneficient because the clients must establish a new TCP, and possibly a new TLS session, for each request. It is understandable considering that the server cannot handle multiple requests in parallel, so you don't want a single client to connect to the server and send requests to it forever and block all the other clients.
The image below shows just how inneficient this is by showing a connection to an HTTPS server on a NodeMCU running at 160MHz with an encryption key of 512bits.
This gets even worse when the encryption key is 2048bits as you can see below.
These two images show how most of the delay is caused by the TLS setup is. This delay can get worse because the TLS setup is also highly dependent on the latency because there's a lot of back and forth.
By reusing the same connection for multiple subsequent requests, we can get rid of the TLS setup for all the subsequent requests and see a great performance boost. There will also be a performance boost for unencrypted requests, but it won't be as significant.
To prevent a client to block all the other clients, I suggest to only allow the current client to stay connected if no other clients are waiting.
I was going to do it. It's just that I prefer to always create an issue so that someone else can work on a solution to the problem if my pull request doesn't get accepted.
Basic Infos
Platform
Settings in IDE
Problem Description
The HTTP server always closes connections to it after 2s (
HTTP_MAX_CLOSE_WAIT
) and never accepts many requests from the same socket. It always returns the headerConnection: close
to tell the client to close the connection.This is extremely inneficient because the clients must establish a new TCP, and possibly a new TLS session, for each request. It is understandable considering that the server cannot handle multiple requests in parallel, so you don't want a single client to connect to the server and send requests to it forever and block all the other clients.
The image below shows just how inneficient this is by showing a connection to an HTTPS server on a NodeMCU running at 160MHz with an encryption key of 512bits.
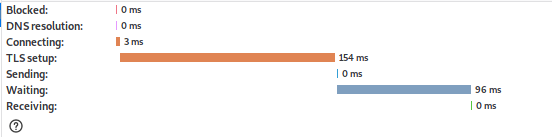
This gets even worse when the encryption key is 2048bits as you can see below.
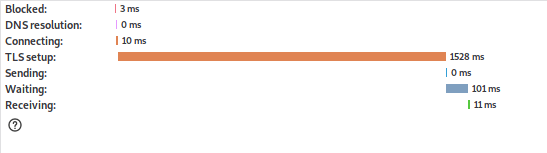
These two images show how most of the delay is caused by the TLS setup is. This delay can get worse because the TLS setup is also highly dependent on the latency because there's a lot of back and forth.
By reusing the same connection for multiple subsequent requests, we can get rid of the TLS setup for all the subsequent requests and see a great performance boost. There will also be a performance boost for unencrypted requests, but it won't be as significant.
To prevent a client to block all the other clients, I suggest to only allow the current client to stay connected if no other clients are waiting.
MCVE Sketch
Benchmarking the solution
I've created a script in ruby that should be helpful to benchmark the solution:
If you change the
DOMAIN
variable togoogle.com
, the result is:We can see the performance gained by keeping the connection alive.
If you change the
DOMAIN
variable tothe IP of your controller
, the result is:We can see that there is currently no performance gain by keeping the connection alive.
Debug Messages
n/a
The text was updated successfully, but these errors were encountered: