Commit 1b95e5d
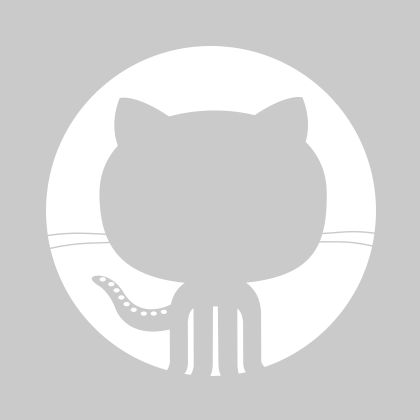
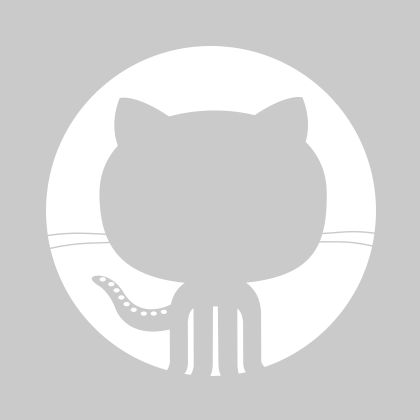
Earle F. Philhower, III
Earle F. Philhower, III
1 parent 4556403 commit 1b95e5d
File tree
10 files changed
+71
-28
lines changed- cores/esp8266
- doc
- libraries
- SD/src
- SDFS/src
- esp8266
- tests/host/fs
10 files changed
+71
-28
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
220 | 220 |
| |
221 | 221 |
| |
222 | 222 |
| |
223 |
| - | |
| 223 | + | |
224 | 224 |
| |
225 | 225 |
| |
226 | 226 |
| |
227 |
| - | |
| 227 | + | |
228 | 228 |
| |
229 | 229 |
| |
230 | 230 |
| |
|
+12-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
119 | 119 |
| |
120 | 120 |
| |
121 | 121 |
| |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
122 | 132 |
| |
123 | 133 |
| |
124 | 134 |
| |
125 | 135 |
| |
126 | 136 |
| |
127 |
| - | |
| 137 | + | |
128 | 138 |
| |
129 | 139 |
| |
130 | 140 |
| |
| |||
166 | 176 |
| |
167 | 177 |
| |
168 | 178 |
| |
| 179 | + | |
169 | 180 |
| |
170 | 181 |
| |
171 | 182 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
69 | 69 |
| |
70 | 70 |
| |
71 | 71 |
| |
72 |
| - | |
| 72 | + | |
73 | 73 |
| |
74 | 74 |
| |
75 | 75 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
136 | 136 |
| |
137 | 137 |
| |
138 | 138 |
| |
139 |
| - | |
| 139 | + | |
140 | 140 |
| |
141 | 141 |
| |
142 | 142 |
| |
| |||
152 | 152 |
| |
153 | 153 |
| |
154 | 154 |
| |
155 |
| - | |
156 |
| - | |
157 |
| - | |
| 155 | + | |
| 156 | + | |
| 157 | + | |
| 158 | + | |
| 159 | + | |
| 160 | + | |
| 161 | + | |
| 162 | + | |
158 | 163 |
| |
159 | 164 |
| |
160 |
| - | |
161 | 165 |
| |
162 | 166 |
| |
163 | 167 |
| |
|
+11-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
134 | 134 |
| |
135 | 135 |
| |
136 | 136 |
| |
137 |
| - | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + | |
| 142 | + | |
| 143 | + | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
| 147 | + | |
138 | 148 |
| |
139 | 149 |
| |
140 | 150 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
32 | 32 |
| |
33 | 33 |
| |
34 | 34 |
| |
35 |
| - | |
36 |
| - | |
| 35 | + | |
| 36 | + | |
37 | 37 |
| |
38 | 38 |
| |
39 | 39 |
| |
| |||
44 | 44 |
| |
45 | 45 |
| |
46 | 46 |
| |
47 |
| - | |
48 |
| - | |
| 47 | + | |
49 | 48 |
| |
50 | 49 |
| |
51 | 50 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
34 | 34 |
| |
35 | 35 |
| |
36 | 36 |
| |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 | 37 |
| |
43 | 38 |
| |
44 | 39 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
44 | 56 |
| |
45 | 57 |
| |
46 | 58 |
| |
| |||
86 | 98 |
| |
87 | 99 |
| |
88 | 100 |
| |
89 |
| - | |
| 101 | + | |
90 | 102 |
| |
91 | 103 |
| |
92 | 104 |
| |
93 |
| - | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
94 | 114 |
| |
95 |
| - | |
96 |
| - | |
97 | 115 |
| |
98 | 116 |
| |
99 | 117 |
| |
| |||
347 | 365 |
| |
348 | 366 |
| |
349 | 367 |
| |
350 |
| - | |
| 368 | + | |
351 | 369 |
| |
352 | 370 |
| |
353 | 371 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
93 | 93 |
| |
94 | 94 |
| |
95 | 95 |
| |
| 96 | + | |
96 | 97 |
| |
97 | 98 |
| |
98 | 99 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
191 | 191 |
| |
192 | 192 |
| |
193 | 193 |
| |
194 |
| - | |
195 | 194 |
| |
| 195 | + | |
| 196 | + | |
196 | 197 |
| |
197 |
| - | |
| 198 | + | |
198 | 199 |
| |
199 | 200 |
| |
200 | 201 |
| |
| |||
207 | 208 |
| |
208 | 209 |
| |
209 | 210 |
| |
210 |
| - | |
| 211 | + | |
| 212 | + | |
| 213 | + | |
211 | 214 |
| |
212 | 215 |
| |
213 | 216 |
| |
| |||
243 | 246 |
| |
244 | 247 |
| |
245 | 248 |
| |
246 |
| - | |
| 249 | + | |
| 250 | + | |
| 251 | + | |
247 | 252 |
| |
248 | 253 |
| |
249 | 254 |
| |
|
0 commit comments