You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
feat(cloudfront-origins): allow custom originPath for apigateway.RestApi constructs (#24023)
### Current behavior
- the `apigateway.RestApi` splits the `restApi.url` and uses the stage name as `originPath` / "Origin path - optional" option in the CloudFront Origin by default
### Issue / Limitation
- when using multiple Behaviors in my CloudFront Distribution:
- S3 as the default behavior
- Regional API Gateway as additional behavior and CloudFront Origin
My API Gateway receives an extra `/<api-gateway-stage>/` in its event path and doesn't trigger the correct lambda integration.
The below CDK example reproduces the behavior mentioned above:
```typescript
import * as cdk from "aws-cdk-lib";
const meLambda = new cdk.aws_lambda_nodejs.NodejsFunction(
this,
"meLambda",
{
entry: "handlers/me.ts",
}
);
const s3_bucket = new cdk.aws_s3.Bucket(this, "somebucket");
const api = new cdk.aws_apigateway.RestApi(this, "somerestapi", {
endpointConfiguration: {
types: [apigw.EndpointType.REGIONAL],
},
defaultCorsPreflightOptions: {
allowOrigins: ["*"],
},
});
api.root
.addResource("me")
.addMethod("GET", new apigw.LambdaIntegration(meLambda));
const apiOrigin = new cdk.aws_cloudfront_origins.RestApiOrigin(api);
const cdn = new cdk.aws_cloudfront.Distribution(this, "websitecdn", {
defaultBehavior: {
origin: new cdk.aws_cloudfront_origins.S3Origin(s3_bucket),
allowedMethods: cdk.aws_cloudfront.AllowedMethods.ALLOW_GET_HEAD_OPTIONS,
viewerProtocolPolicy: cdk.aws_cloudfront.ViewerProtocolPolicy.REDIRECT_TO_HTTPS,
},
additionalBehaviors: {
"prod/*": { // <<<---- The "prod/" is added in the CloudFront Origin Path, NOT DESIRED IS THIS CASE
origin: apiOrigin,
allowedMethods: cdk.aws_cloudfront.AllowedMethods.ALLOW_ALL,
cachePolicy: cdk.aws_cloudfront.CachePolicy.CACHING_DISABLED,
viewerProtocolPolicy: cdk.aws_cloudfront.ViewerProtocolPolicy.HTTPS_ONLY,
},
},
});
```
The above will have the following URLs:
- `https://<api-gateway-url>/<api-gateway-stage>`
- `https://<cloudfront-distribution-url>/some-image.png` => Default Behavior, S3 Bucket
- `https://<cloudfront-distribution-url>/<api-gateway-stage>` => API Gateway forward request
Because the CloudFront Origin created by `RestApiOrigin` appends the `<api-gateway-stage>` in its CloudFront Origin "Origin Path - optional" option, when using `https://<cloudfront-distribution-url>/<api-gateway-stage>/me` the API Gateway receives `/<api-gateway-stage>/<api-gateway-stage>/me` instead of `/<api-gateway-stage>/me`
Removing the "Origin Path - optional" value, fixes the problem.
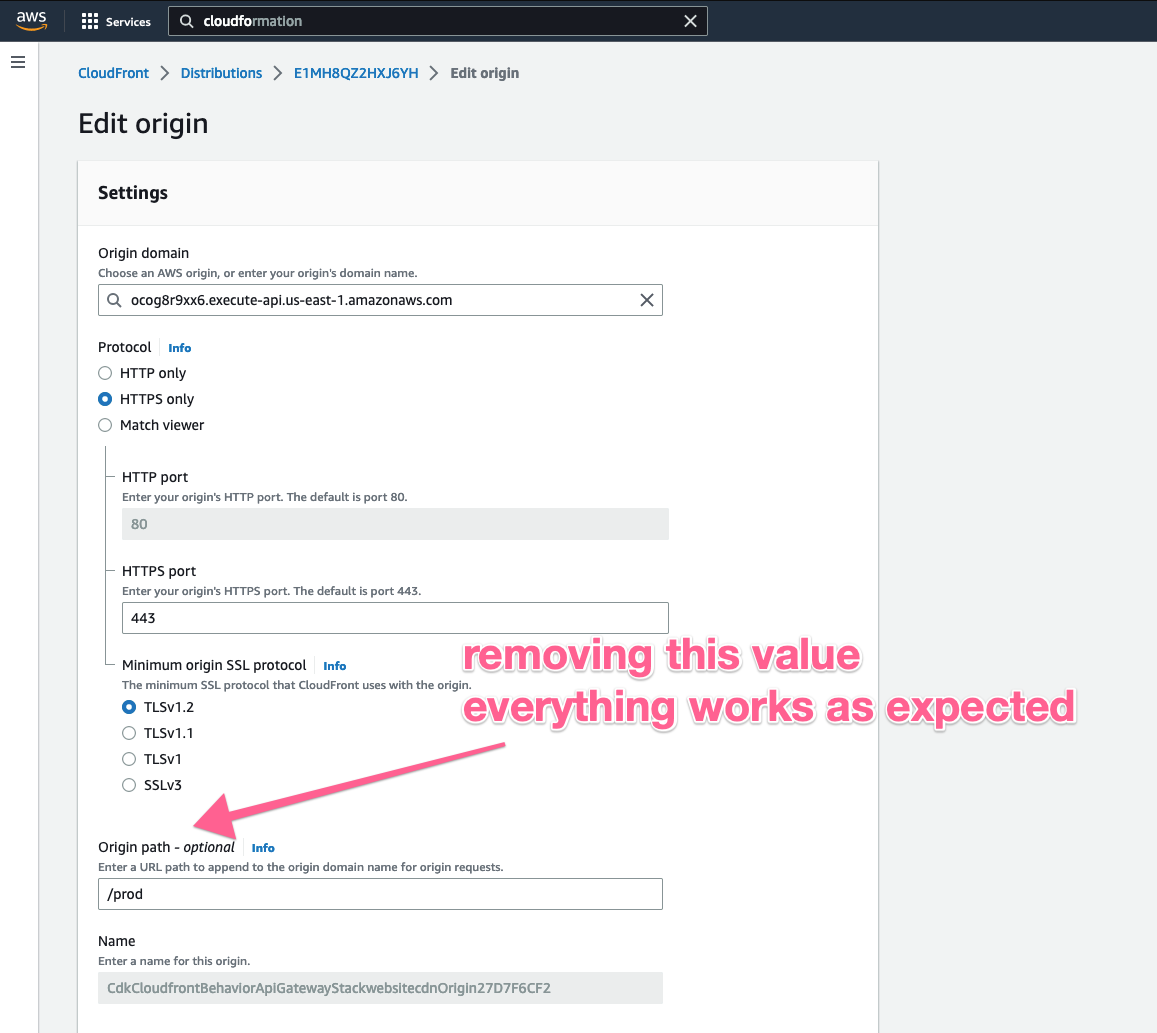
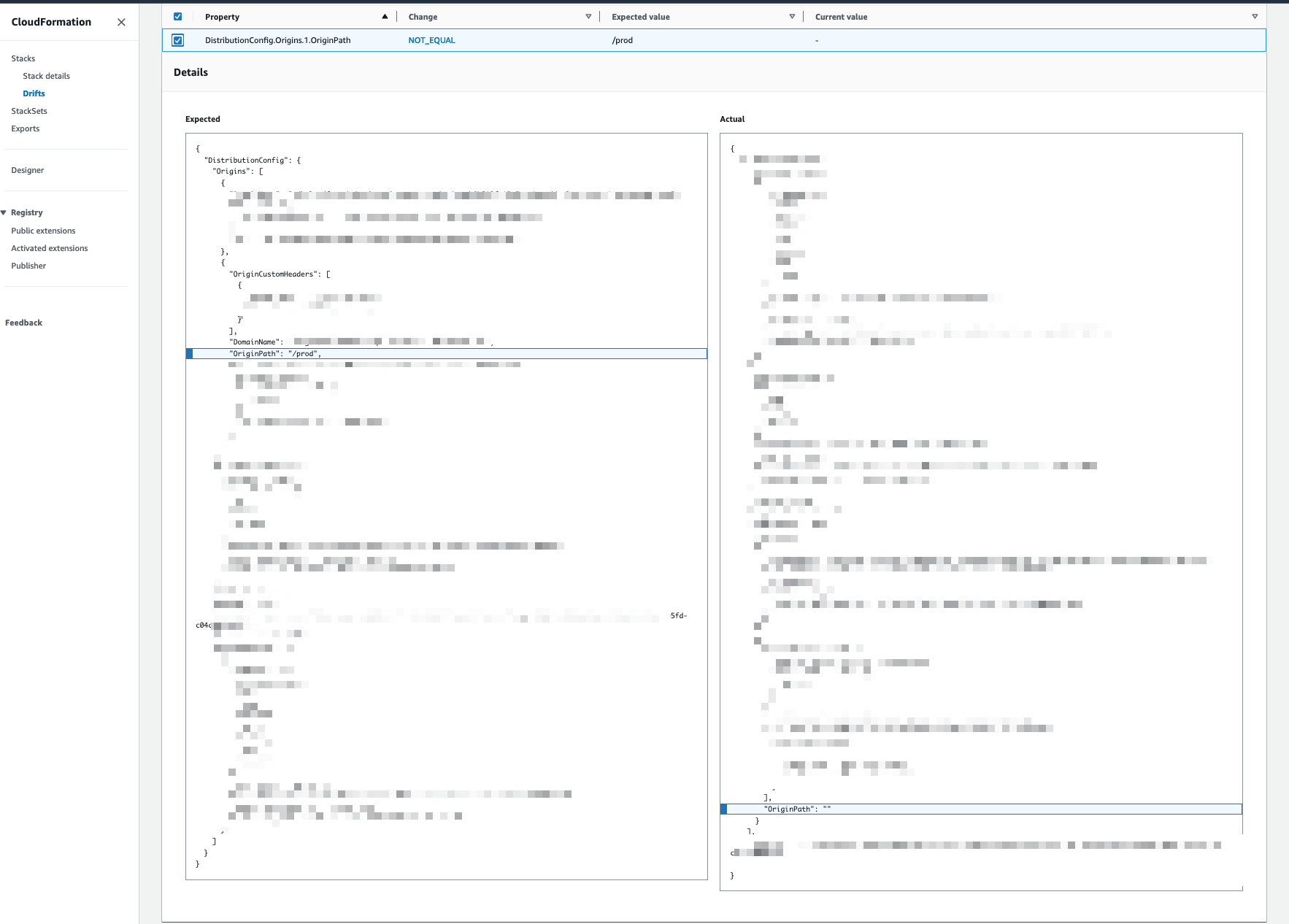
### Workaround
- To fix the problem described above, I need to use a custom `cdk.aws_cloudfront_origins.HttpOrigin` construct
Replace the `apiOrigin` construct in the example above by:
```typescript
const apiOrigin = new origins.HttpOrigin(
`${api.restApiId}.execute-api.${cdk.Aws.REGION}.${cdk.Aws.URL_SUFFIX}`,
{
originSslProtocols: [cloudfront.OriginSslPolicy.TLS_V1_2],
protocolPolicy: cloudfront.OriginProtocolPolicy.HTTPS_ONLY,
}
);
```
### Solution
- This PR allows the customization of `apigateway.RestApi` by extending its props from `cloudfront.OriginProps`
- Allowing the consumer to pass a `props.originPath` to the `RestApiOrigin` class
Copy file name to clipboardExpand all lines: packages/@aws-cdk/aws-cloudfront-origins/README.md
+9-2
Original file line number
Diff line number
Diff line change
@@ -122,7 +122,7 @@ new cloudfront.Distribution(this, 'myDist', {
122
122
## From an API Gateway REST API
123
123
124
124
Origins can be created from an API Gateway REST API. It is recommended to use a
125
-
[regional API](https://docs.aws.amazon.com/apigateway/latest/developerguide/api-gateway-api-endpoint-types.html) in this case.
125
+
[regional API](https://docs.aws.amazon.com/apigateway/latest/developerguide/api-gateway-api-endpoint-types.html) in this case. The origin path will automatically be set as the stage name.
126
126
127
127
```ts
128
128
declareconst api:apigateway.RestApi;
@@ -131,4 +131,11 @@ new cloudfront.Distribution(this, 'Distribution', {
131
131
});
132
132
```
133
133
134
-
The origin path will automatically be set as the stage name.
134
+
If you want to use a different origin path, you can specify it in the `originPath` property.
0 commit comments