You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Copy file name to clipboardExpand all lines: packages/@aws-cdk/aws-pipes-alpha/README.md
+33-106
Original file line number
Diff line number
Diff line change
@@ -18,14 +18,12 @@
18
18
19
19
20
20
EventBridge Pipes let you create source to target connections between several
21
-
aws services. While transporting messages from a source to a target the messages
21
+
AWS services. While transporting messages from a source to a target the messages
22
22
can be filtered, transformed and enriched.
23
23
24
24
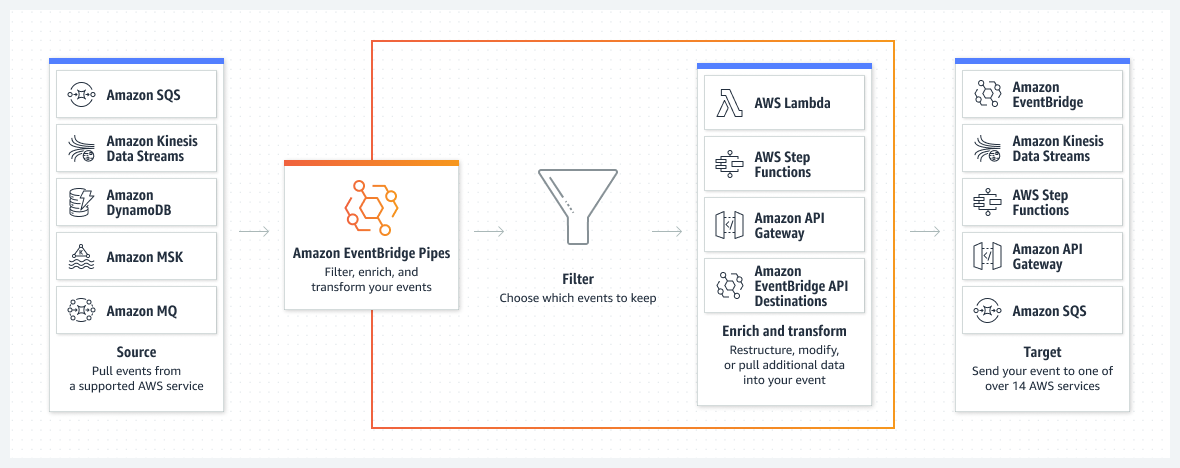
For more details see the [service documentation](https://docs.aws.amazon.com/eventbridge/latest/userguide/eb-pipes.html).
29
27
30
28
## Pipe
31
29
@@ -34,12 +32,12 @@ is a fully managed service that enables point-to-point integrations between
34
32
event producers and consumers. Pipes can be used to connect several AWS services
35
33
to each other, or to connect AWS services to external services.
36
34
37
-
A Pipe has a source and a target. The source events can be filtered and enriched
35
+
A pipe has a source and a target. The source events can be filtered and enriched
38
36
before reaching the target.
39
37
40
38
## Example - pipe usage
41
39
42
-
> The following code examples use an example implementation of a [source](#source) and [target](#target). In the future there will be separate packages for the sources and targets.
40
+
> The following code examples use an example implementation of a [source](#source) and [target](#target).
43
41
44
42
To define a pipe you need to create a new `Pipe` construct. The `Pipe` construct needs a source and a target.
45
43
@@ -58,49 +56,30 @@ Messages from the source are put into the body of the target message.
58
56
59
57
## Source
60
58
61
-
A source is a AWS Service that is polled. The following Sources are
62
-
possible:
59
+
A source is a AWS Service that is polled. The following sources are possible:
> Currently no implementation exist for any of the supported sources. The following example shows how an implementation can look like. The actual implementation is not part of this package and will be in a separate one.
68
+
Currently, DynamoDB, Kinesis, and SQS are supported. If you are interested in support for additional sources,
69
+
kindly let us know by opening a GitHub issue or raising a PR.
72
70
73
-
### Example source implementation
71
+
### Example source
74
72
75
-
```ts fixture=pipes-imports
76
-
classSqsSourceimplementspipes.ISource {
77
-
sourceArn:string;
78
-
sourceParameters =undefined;
79
-
80
-
constructor(privatereadonlyqueue:sqs.Queue) {
81
-
this.queue=queue;
82
-
this.sourceArn=queue.queueArn;
83
-
}
84
-
85
-
bind(_pipe:pipes.IPipe):pipes.SourceConfig {
86
-
return {
87
-
sourceParameters: this.sourceParameters,
88
-
};
89
-
}
90
-
91
-
grantRead(pipeRole:cdk.aws_iam.IRole):void {
92
-
this.queue.grantConsumeMessages(pipeRole);
93
-
}
94
-
}
73
+
```ts
74
+
declareconst sourceQueue:sqs.Queue;
75
+
const pipeSource =newSqsSource(sourceQueue);
95
76
```
96
77
97
-
A source implementation needs to provide the `sourceArn`, `sourceParameters` and grant the pipe role read access to the source.
98
-
99
78
## Filter
100
79
101
-
A Filter can be used to filter the events from the source before they are
80
+
A filter can be used to filter the events from the source before they are
102
81
forwarded to the enrichment or, if no enrichment is present, target step. Multiple filter expressions are possible.
103
-
If one of the filter expressions matches the event is forwarded to the enrichment or target step.
82
+
If one of the filter expressions matches, the event is forwarded to the enrichment or target step.
104
83
105
84
### Example - filter usage
106
85
@@ -130,7 +109,7 @@ This example shows a filter that only forwards events with the `customerType` B2
130
109
131
110
You can define multiple filter pattern which are combined with a logical `OR`.
132
111
133
-
Additional filter pattern and details can be found in the EventBridge pipes [docs](https://docs.aws.amazon.com/eventbridge/latest/userguide/eb-pipes-event-filtering.html)
112
+
Additional filter pattern and details can be found in the EventBridge pipes [docs](https://docs.aws.amazon.com/eventbridge/latest/userguide/eb-pipes-event-filtering.html).
134
113
135
114
## Input transformation
136
115
@@ -175,7 +154,7 @@ So when the following batch of input events is processed by the pipe
175
154
]
176
155
```
177
156
178
-
it is converted into the following payload.
157
+
it is converted into the following payload:
179
158
180
159
```json
181
160
[
@@ -189,7 +168,7 @@ it is converted into the following payload.
189
168
]
190
169
```
191
170
192
-
If the transformation is applied to a target it might be converted to a string representation. E.g. the resulting SQS message body looks like this.
171
+
If the transformation is applied to a target it might be converted to a string representation. For example, the resulting SQS message body looks like this:
193
172
194
173
```json
195
174
[
@@ -237,7 +216,7 @@ So when the following batch of input events is processed by the pipe
237
216
]
238
217
```
239
218
240
-
it is converted into the following target payload.
219
+
it is converted into the following target payload:
241
220
242
221
```json
243
222
[
@@ -283,8 +262,7 @@ This transformation forwards the static text to the target.
283
262
284
263
## Enrichment
285
264
286
-
In the enrichment step the (un)filtered payloads from the source can be used to
287
-
invoke one of the following services
265
+
In the enrichment step the (un)filtered payloads from the source can be used to invoke one of the following services:
288
266
289
267
- API destination
290
268
- Amazon API Gateway
@@ -420,95 +398,44 @@ targets are supported:
420
398
The target event can be transformed before it is forwarded to the target using
421
399
the same input transformation as in the enrichment step.
422
400
423
-
### Example target implementation
424
-
425
-
> Currently no implementation exist for any of the supported targets. The following example shows how an implementation can look like. The actual implementation is not part of this package and will be in a separate one.
A target implementation needs to provide the `targetArn`, `enrichmentParameters` and grant the pipe role invoke access to the enrichment.
453
-
454
408
## Log destination
455
409
456
410
A pipe can produce log events that are forwarded to different log destinations.
457
411
You can configure multiple destinations, but all the destination share the same log level and log data.
458
412
For details check the official [documentation](https://docs.aws.amazon.com/eventbridge/latest/userguide/eb-pipes-logs.html).
459
413
460
414
The log level and data that is included in the log events is configured on the pipe class itself.
461
-
Whereas the actual destination is defined independent.
462
-
463
-
### Example log destination implementation
415
+
The actual destination is defined independently, and there are three options:
464
416
465
-
> Currently no implementation exist for any of the supported enrichments. The following example shows how an implementation can look like. The actual implementation is not part of this package and will be in a separate one.
This example uses a cloudwatch loggroup to store the log emitted during a pipe execution. The log level is set to `TRACE` so all steps of the pipe are logged.
439
+
This example uses a CloudWatch Logs log group to store the log emitted during a pipe execution.
440
+
The log level is set to `TRACE` so all steps of the pipe are logged.
514
441
Additionally all execution data is logged as well.
0 commit comments