|
| 1 | +[](https://github.com/LeetCode-in-TypeScript/LeetCode-in-TypeScript) |
| 2 | +[](https://github.com/LeetCode-in-TypeScript/LeetCode-in-TypeScript/fork) |
| 3 | + |
| 4 | +## 437\. Path Sum III |
| 5 | + |
| 6 | +Medium |
| 7 | + |
| 8 | +Given the `root` of a binary tree and an integer `targetSum`, return _the number of paths where the sum of the values along the path equals_ `targetSum`. |
| 9 | + |
| 10 | +The path does not need to start or end at the root or a leaf, but it must go downwards (i.e., traveling only from parent nodes to child nodes). |
| 11 | + |
| 12 | +**Example 1:** |
| 13 | + |
| 14 | +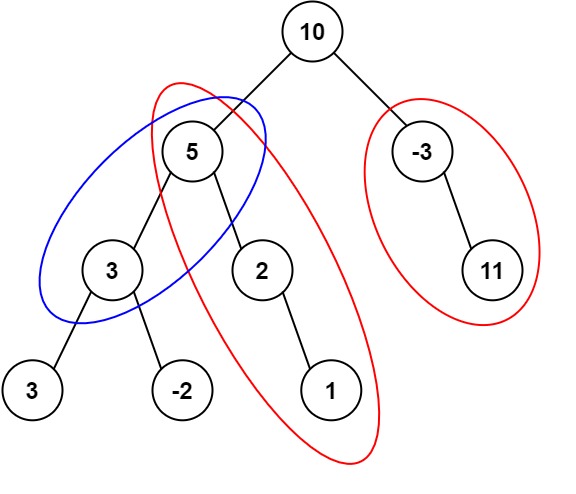 |
| 15 | + |
| 16 | +**Input:** root = [10,5,-3,3,2,null,11,3,-2,null,1], targetSum = 8 |
| 17 | + |
| 18 | +**Output:** 3 |
| 19 | + |
| 20 | +**Explanation:** The paths that sum to 8 are shown. |
| 21 | + |
| 22 | +**Example 2:** |
| 23 | + |
| 24 | +**Input:** root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22 |
| 25 | + |
| 26 | +**Output:** 3 |
| 27 | + |
| 28 | +**Constraints:** |
| 29 | + |
| 30 | +* The number of nodes in the tree is in the range `[0, 1000]`. |
| 31 | +* <code>-10<sup>9</sup> <= Node.val <= 10<sup>9</sup></code> |
| 32 | +* `-1000 <= targetSum <= 1000` |
| 33 | + |
| 34 | +## Solution |
| 35 | + |
| 36 | +```typescript |
| 37 | +function pathSum(root: TreeNode | null, targetSum: number): number { |
| 38 | + let count = 0 |
| 39 | + let map = new Map<number, number>() |
| 40 | + |
| 41 | + function dfs(node: TreeNode | null, currentSum: number): void { |
| 42 | + if (!node) return |
| 43 | + |
| 44 | + currentSum += node.val |
| 45 | + if (currentSum === targetSum) count++ |
| 46 | + |
| 47 | + count += map.get(currentSum - targetSum) ?? 0 |
| 48 | + |
| 49 | + map.set(currentSum, map.get(currentSum) + 1 || 1) |
| 50 | + dfs(node?.left, currentSum) |
| 51 | + dfs(node?.right, currentSum) |
| 52 | + |
| 53 | + //remove from hashmap |
| 54 | + map.set(currentSum, map.get(currentSum) - 1) |
| 55 | + if (map.get(currentSum) === 0) map.delete(currentSum) |
| 56 | + } |
| 57 | + |
| 58 | + dfs(root, 0) |
| 59 | + return count |
| 60 | +} |
| 61 | + |
| 62 | +export { pathSum } |
| 63 | +``` |
0 commit comments