|
| 1 | +# 2196. Create Binary Tree From Descriptions |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Array, Hash Table, Tree, Depth-First Search, Breadth-First Search, Binary Tree. |
| 5 | +- Similar Questions: Convert Sorted List to Binary Search Tree, Number Of Ways To Reconstruct A Tree. |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +You are given a 2D integer array `descriptions` where `descriptions[i] = [parenti, childi, isLefti]` indicates that `parenti` is the **parent** of `childi` in a **binary** tree of **unique** values. Furthermore, |
| 10 | + |
| 11 | + |
| 12 | + |
| 13 | +- If `isLefti == 1`, then `childi` is the left child of `parenti`. |
| 14 | + |
| 15 | +- If `isLefti == 0`, then `childi` is the right child of `parenti`. |
| 16 | + |
| 17 | + |
| 18 | +Construct the binary tree described by `descriptions` and return **its **root****. |
| 19 | + |
| 20 | +The test cases will be generated such that the binary tree is **valid**. |
| 21 | + |
| 22 | + |
| 23 | +Example 1: |
| 24 | + |
| 25 | +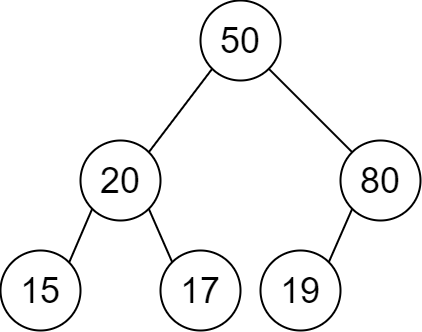 |
| 26 | + |
| 27 | +``` |
| 28 | +Input: descriptions = [[20,15,1],[20,17,0],[50,20,1],[50,80,0],[80,19,1]] |
| 29 | +Output: [50,20,80,15,17,19] |
| 30 | +Explanation: The root node is the node with value 50 since it has no parent. |
| 31 | +The resulting binary tree is shown in the diagram. |
| 32 | +``` |
| 33 | + |
| 34 | +Example 2: |
| 35 | + |
| 36 | +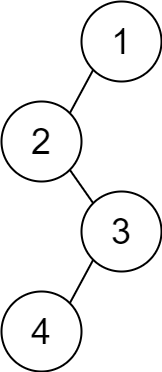 |
| 37 | + |
| 38 | +``` |
| 39 | +Input: descriptions = [[1,2,1],[2,3,0],[3,4,1]] |
| 40 | +Output: [1,2,null,null,3,4] |
| 41 | +Explanation: The root node is the node with value 1 since it has no parent. |
| 42 | +The resulting binary tree is shown in the diagram. |
| 43 | +``` |
| 44 | + |
| 45 | + |
| 46 | +**Constraints:** |
| 47 | + |
| 48 | + |
| 49 | + |
| 50 | +- `1 <= descriptions.length <= 104` |
| 51 | + |
| 52 | +- `descriptions[i].length == 3` |
| 53 | + |
| 54 | +- `1 <= parenti, childi <= 105` |
| 55 | + |
| 56 | +- `0 <= isLefti <= 1` |
| 57 | + |
| 58 | +- The binary tree described by `descriptions` is valid. |
| 59 | + |
| 60 | + |
| 61 | + |
| 62 | +## Solution |
| 63 | + |
| 64 | +```javascript |
| 65 | +/** |
| 66 | + * Definition for a binary tree node. |
| 67 | + * function TreeNode(val, left, right) { |
| 68 | + * this.val = (val===undefined ? 0 : val) |
| 69 | + * this.left = (left===undefined ? null : left) |
| 70 | + * this.right = (right===undefined ? null : right) |
| 71 | + * } |
| 72 | + */ |
| 73 | +/** |
| 74 | + * @param {number[][]} descriptions |
| 75 | + * @return {TreeNode} |
| 76 | + */ |
| 77 | +var createBinaryTree = function(descriptions) { |
| 78 | + var nodeMap = {}; |
| 79 | + var hasNoParentMap = {}; |
| 80 | + for (var i = 0; i < descriptions.length; i++) { |
| 81 | + var [parent, child, isLeft] = descriptions[i]; |
| 82 | + if (hasNoParentMap[parent] === undefined) hasNoParentMap[parent] = true; |
| 83 | + hasNoParentMap[child] = false; |
| 84 | + if (!nodeMap[parent]) nodeMap[parent] = new TreeNode(parent); |
| 85 | + if (!nodeMap[child]) nodeMap[child] = new TreeNode(child); |
| 86 | + if (isLeft) { |
| 87 | + nodeMap[parent].left = nodeMap[child]; |
| 88 | + } else { |
| 89 | + nodeMap[parent].right = nodeMap[child]; |
| 90 | + } |
| 91 | + } |
| 92 | + return nodeMap[Object.keys(hasNoParentMap).filter(key => hasNoParentMap[key])[0]]; |
| 93 | +}; |
| 94 | +``` |
| 95 | + |
| 96 | +**Explain:** |
| 97 | + |
| 98 | +nope. |
| 99 | + |
| 100 | +**Complexity:** |
| 101 | + |
| 102 | +* Time complexity : O(n). |
| 103 | +* Space complexity : O(n). |
0 commit comments