|
| 1 | +# 1424. Diagonal Traverse II |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Array, Sorting, Heap (Priority Queue). |
| 5 | +- Similar Questions: . |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +Given a 2D integer array `nums`, return **all elements of **`nums`** in diagonal order as shown in the below images**. |
| 10 | + |
| 11 | + |
| 12 | +Example 1: |
| 13 | + |
| 14 | +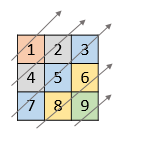 |
| 15 | + |
| 16 | +``` |
| 17 | +Input: nums = [[1,2,3],[4,5,6],[7,8,9]] |
| 18 | +Output: [1,4,2,7,5,3,8,6,9] |
| 19 | +``` |
| 20 | + |
| 21 | +Example 2: |
| 22 | + |
| 23 | +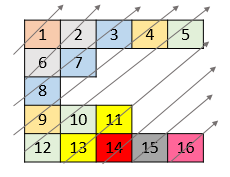 |
| 24 | + |
| 25 | +``` |
| 26 | +Input: nums = [[1,2,3,4,5],[6,7],[8],[9,10,11],[12,13,14,15,16]] |
| 27 | +Output: [1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16] |
| 28 | +``` |
| 29 | + |
| 30 | + |
| 31 | +**Constraints:** |
| 32 | + |
| 33 | + |
| 34 | + |
| 35 | +- `1 <= nums.length <= 105` |
| 36 | + |
| 37 | +- `1 <= nums[i].length <= 105` |
| 38 | + |
| 39 | +- `1 <= sum(nums[i].length) <= 105` |
| 40 | + |
| 41 | +- `1 <= nums[i][j] <= 105` |
| 42 | + |
| 43 | + |
| 44 | + |
| 45 | +## Solution |
| 46 | + |
| 47 | +```javascript |
| 48 | +/** |
| 49 | + * @param {number[][]} nums |
| 50 | + * @return {number[]} |
| 51 | + */ |
| 52 | +var findDiagonalOrder = function(nums) { |
| 53 | + var groups = []; |
| 54 | + for (var i = nums.length - 1 ; i >= 0; i--) { |
| 55 | + for (var j = 0; j < nums[i].length; j++) { |
| 56 | + groups[i + j] = groups[i + j] || []; |
| 57 | + groups[i + j].push(nums[i][j]); |
| 58 | + } |
| 59 | + } |
| 60 | + return [].concat(...groups); |
| 61 | +}; |
| 62 | +``` |
| 63 | + |
| 64 | +**Explain:** |
| 65 | + |
| 66 | +nope. |
| 67 | + |
| 68 | +**Complexity:** |
| 69 | + |
| 70 | +* Time complexity : O(n). |
| 71 | +* Space complexity : O(n). |
0 commit comments